Introduction to Python II#
In today’s workshop we will learn about Python functions and popular Libraries: Numpy and Matplotlib
#import antigravity
#library --> collection of functions
#function --> collection of instructions to perform a task/s
Workshop Setup#
import random
import numpy as np
import matplotlib.pyplot as plt
---------------------------------------------------------------------------
ImportError Traceback (most recent call last)
Cell In[2], line 3
1 import random
2 import numpy as np
----> 3 import matplotlib.pyplot as plt
File ~/.conda/envs/jupyter-book/lib/python3.10/site-packages/matplotlib/__init__.py:161
157 from packaging.version import parse as parse_version
159 # cbook must import matplotlib only within function
160 # definitions, so it is safe to import from it here.
--> 161 from . import _api, _version, cbook, _docstring, rcsetup
162 from matplotlib._api import MatplotlibDeprecationWarning
163 from matplotlib.rcsetup import cycler # noqa: F401
File ~/.conda/envs/jupyter-book/lib/python3.10/site-packages/matplotlib/cbook.py:32
29 from numpy import VisibleDeprecationWarning
31 import matplotlib
---> 32 from matplotlib import _api, _c_internal_utils
35 class _ExceptionInfo:
36 """
37 A class to carry exception information around.
38
(...)
43 users and result in incorrect tracebacks.
44 """
ImportError: /lib64/libstdc++.so.6: version `GLIBCXX_3.4.29' not found (required by /home/cehrett/.conda/envs/jupyter-book/lib/python3.10/site-packages/matplotlib/_c_internal_utils.cpython-310-x86_64-linux-gnu.so)
Functions#
Creating a function from scratch#
A simple function#
Step 1:#
Defining a function
def cheerup():
print("Good Morning!")
cheerup()
Good Morning!
Step 2:#
Adding a comment
def cheerup():
#Prints a greeting
print("Good Morning!")
Step 3:#
Adding a Docstring
def cheerup(name = "Tigers"):
#Prints a greeting
'''Prints a greeting for the user'''
print("Good Morning!")
cheerup?
Step 4:#
Passing Arguments
def cheerup(name):
#Prints a greeting
'''Prints a greeting for the user'''
print(f"Good Morning {name}!!")
cheerup("Monty")
Good Morning Monty!!
cheerup()
---------------------------------------------------------------------------
TypeError Traceback (most recent call last)
/var/folders/2g/16vjth1s5y38rsg8fh0yt1_40000gp/T/ipykernel_75125/1191407940.py in <module>
----> 1 cheerup()
TypeError: cheerup() missing 1 required positional argument: 'name'
Step 5:#
Adding a default Argument
def cheerup(name = "Tigers"):
#Prints a greeting
'''Prints a greeting for the user'''
print(f"Good Morning {name}!!")
cheerup() # Uses default value for argument
Good Morning Tigers!!
cheerup("Monty")
Good Morning Monty!!
cheerup(3.14)
Good Morning 3.14!!
Recap: Lists#
#2D arrays --> List of lists
a = [[1,2,3],
[2,3,4],
[5,6,7],
[5,8,9]]
b = [[1,2,3,4],
[5,6,7,8],
[9,10,11,12]]
type(a[0])
list
len(b[0])
4
Recap: For loops, List Comprehensions#
for num in range(4):
print(num)
0
1
2
3
[0 for row in range(4)]
[0, 0, 0, 0]
[[0 for col in range(4)] for row in range(4)]
[[0, 0, 0, 0], [0, 0, 0, 0], [0, 0, 0, 0], [0, 0, 0, 0]]
test = [[0 for col in range(4)] for row in range(4)]
test #initializing a matrix/2D-Array to zero
[[0, 0, 0, 0], [0, 0, 0, 0], [0, 0, 0, 0], [0, 0, 0, 0]]
Creating Matrix with Random numbers#
random.random()
0.5889256775431918
#Using List Comprehensions
m = 5 #Row size
n = 4 #Column size
[[random.random() for col in range(n)] for row in range(m)]
[[0.01904841858110551,
0.40856794843651256,
0.4237015344083579,
0.5159002870954503],
[0.19188830987094907,
0.6406836577700511,
0.8441107563371169,
0.5448175231004886],
[0.4402972073918322,
0.29580813089509783,
0.3416911455699272,
0.5746066321486847],
[0.1884536428513407,
0.5223120232280826,
0.5126194623515107,
0.2737934421533338],
[0.45516446819413614,
0.29316264120710533,
0.9209667766637216,
0.8742949769692125]]
##Creating a function to generate random matrices
def random_matrix(m,n):
out = [[random.random() for col in range(n)] for row in range(m)]
##return the Random Matrix to be used elsewhere
##in th eprogram
return out
random_matrix(4,3)
[[0.4360689094065805, 0.900394993531916, 0.4067655965463516],
[0.1470437037432799, 0.6906740887550721, 0.029994897208805105],
[0.9067333996658661, 0.8690265862176723, 0.6744283473171534],
[0.2969922994198114, 0.9615761616182039, 0.8131958976252768]]
#function to multiply two matrices
def matmul_custom(A,B):
#Defining the dimension of the result matrix
rows_out = len(A)
cols_out = len(B[0])
#Initializing: assigning zero values
out = [[0 for col in range(cols_out)] for row in range(rows_out)]
for i in range(rows_out):
for j in range(cols_out):
for k in range(len(B)):
out[i][j] = out[i][j]+A[i][k]*B[k][j]
return out
matmul_custom(a,b)
[[38, 44, 50, 56], [53, 62, 71, 80], [98, 116, 134, 152], [126, 148, 170, 192]]
rand_a = random_matrix(400,300)
rand_b = random_matrix(300,400)
%%time
rand_res = matmul_custom(rand_a,rand_b)
CPU times: user 8.66 s, sys: 26.1 ms, total: 8.68 s
Wall time: 8.7 s
Using Numpy#
np.
is a library prefix and helps identify from which library a particular function is being used/called.
For example, the random function exists in the random
library and the numpy
library.
Instead of typing the entire library name, we shorten it so that it is easier to type.
import numpy as np
np.lookfor("matrix multiplication")
Show code cell output
Search results for 'matrix multiplication'
------------------------------------------
numpy.core._umath_tests.matrix_multiply
matrix multiplication on last two dimensions
numpy.matrix
.. note:: It is no longer recommended to use this class, even for linear
numpy.matmul
Matrix product of two arrays.
numpy.linalg.matrix_power
Raise a square matrix to the (integer) power `n`.
numpy.dot
Dot product of two arrays. Specifically,
numpy.einsum
einsum(subscripts, *operands, out=None, dtype=None, order='K',
numpy.vectorize
vectorize(pyfunc, otypes=None, doc=None, excluded=None, cache=False,
numpy.linalg.multi_dot
Compute the dot product of two or more arrays in a single function call,
numpy.core._multiarray_umath.dot
Dot product of two arrays. Specifically,
numpy.core._multiarray_umath.c_einsum
c_einsum(subscripts, *operands, out=None, dtype=None, order='K',
%%time
rand_npres = np.matmul(rand_a, rand_b)
CPU times: user 22.7 ms, sys: 10 ms, total: 32.8 ms
Wall time: 23.3 ms
%timeit rand_npres = np.matmul(rand_a, rand_b)
15.3 ms ± 2.14 ms per loop (mean ± std. dev. of 7 runs, 100 loops each)
Note:
Jupyter/IPython Magic Commands
%%time
is a cell magic command that gives you the total time it took to execute the contents of a cell.
%timeit
is a line magic command that tells you average time it takes to run a line of code.
np.lookfor("random")
Show code cell output
Search results for 'random'
---------------------------
numpy.random.ranf
This is an alias of `random_sample`. See `random_sample` for the complete
numpy.random.PCG64
BitGenerator for the PCG-64 pseudo-random number generator.
numpy.random.Philox
Container for the Philox (4x64) pseudo-random number generator.
numpy.random.sample
This is an alias of `random_sample`. See `random_sample` for the complete
numpy.random.MT19937
Container for the Mersenne Twister pseudo-random number generator.
numpy.random.PCG64DXSM
BitGenerator for the PCG-64 DXSM pseudo-random number generator.
numpy.matlib.rand
Return a matrix of random values with given shape.
numpy.matlib.randn
Return a random matrix with data from the "standard normal" distribution.
numpy.random.RandomState
Container for the slow Mersenne Twister pseudo-random number generator.
numpy.random.Generator.bytes
bytes(length)
numpy.random.Generator.choice
Generates a random sample from a given array
numpy.random.Generator.normal
Draw random samples from a normal (Gaussian) distribution.
numpy.random.Generator.random
Return random floats in the half-open interval [0.0, 1.0).
numpy.random.RandomState.rand
Random values in a given shape.
numpy.random.RandomState.bytes
bytes(length)
numpy.random.Generator.integers
Return random integers from `low` (inclusive) to `high` (exclusive), or
numpy.random.Generator.permuted
Randomly permute `x` along axis `axis`.
numpy.random.RandomState.choice
Generates a random sample from a given 1-D array
numpy.random.RandomState.normal
Draw random samples from a normal (Gaussian) distribution.
numpy.random.RandomState.random
Return random floats in the half-open interval [0.0, 1.0). Alias for
numpy.random.RandomState.randint
Return random integers from `low` (inclusive) to `high` (exclusive).
numpy.random.RandomState.tomaxint
Return a sample of uniformly distributed random integers in the interval
numpy.random.Generator.permutation
Randomly permute a sequence, or return a permuted range.
numpy.random.BitGenerator.random_raw
Return randoms as generated by the underlying BitGenerator
numpy.random.RandomState.permutation
permutation(x)
numpy.random.RandomState.random_sample
Return random floats in the half-open interval [0.0, 1.0).
numpy.random._pickle.__randomstate_ctor
Pickling helper function that returns a legacy RandomState-like object
numpy.random.RandomState.random_integers
Random integers of type `np.int_` between `low` and `high`, inclusive.
numpy.flip
Reverse the order of elements in an array along the given axis.
numpy.poly
Find the coefficients of a polynomial with the given sequence of roots.
numpy.sort
Return a sorted copy of an array.
numpy.stack
Join a sequence of arrays along a new axis.
numpy.random.RandomState.multivariate_normal
Draw random samples from a multivariate normal distribution.
numpy.fliplr
Reverse the order of elements along axis 1 (left/right).
numpy.flipud
Reverse the order of elements along axis 0 (up/down).
numpy.ndarray
ndarray(shape, dtype=float, buffer=None, offset=0,
numpy.convolve
Returns the discrete, linear convolution of two one-dimensional sequences.
numpy.corrcoef
Return Pearson product-moment correlation coefficients.
numpy.histogram
Compute the histogram of a dataset.
numpy.einsum_path
Evaluates the lowest cost contraction order for an einsum expression by
numpy.histogram2d
Compute the bi-dimensional histogram of two data samples.
numpy.histogramdd
Compute the multidimensional histogram of some data.
numpy.random.SFC64
BitGenerator for Chris Doty-Humphrey's Small Fast Chaotic PRNG.
numpy.fft.fftn
Compute the N-dimensional discrete Fourier Transform.
numpy.fft.ifft
Compute the one-dimensional inverse discrete Fourier Transform.
numpy.fft.ifftn
Compute the N-dimensional inverse discrete Fourier Transform.
numpy.ma.stack
Join a sequence of arrays along a new axis.
numpy.linalg.qr
Compute the qr factorization of a matrix.
numpy.linalg.svd
Singular Value Decomposition.
numpy.savez_compressed
Save several arrays into a single file in compressed ``.npz`` format.
numpy.random.Generator
Generator(bit_generator)
numpy.linalg.pinv
Compute the (Moore-Penrose) pseudo-inverse of a matrix.
numpy.matlib.empty
Return a new matrix of given shape and type, without initializing entries.
numpy.chararray.item
a.item(*args)
numpy.random.default_rng
Construct a new Generator with the default BitGenerator (PCG64).
numpy.set_string_function
Set a Python function to be used when pretty printing arrays.
numpy.random.BitGenerator
Base Class for generic BitGenerators, which provide a stream
numpy.random.SeedSequence
SeedSequence mixes sources of entropy in a reproducible way to set the
numpy.linalg.eigvals
Compute the eigenvalues of a general matrix.
numpy.chararray.itemset
a.itemset(*args)
numpy.linalg.multi_dot
Compute the dot product of two or more arrays in a single function call,
numpy.linalg.tensorinv
Compute the 'inverse' of an N-dimensional array.
numpy.random.tests.test_random.TestRandint.rfunc
Return random integers from `low` (inclusive) to `high` (exclusive).
numpy.linalg.tensorsolve
Solve the tensor equation ``a x = b`` for x.
numpy.random.Generator.f
Draw samples from an F distribution.
numpy.distutils.read_config
Return library info for a package from its configuration file.
numpy.random.PCG64.jumped
Returns a new bit generator with the state jumped.
numpy.random.PCG64.advance
advance(delta)
numpy.random.Philox.jumped
Returns a new bit generator with the state jumped
numpy.random.RandomState.f
Draw samples from an F distribution.
numpy.random.Generator.wald
Draw samples from a Wald, or inverse Gaussian, distribution.
numpy.random.Generator.zipf
Draw samples from a Zipf distribution.
numpy.random.MT19937.jumped
Returns a new bit generator with the state jumped
numpy.random.Philox.advance
advance(delta)
numpy.random.Generator.gamma
Draw samples from a Gamma distribution.
numpy.random.Generator.power
Draws samples in [0, 1] from a power distribution with positive
numpy.random.Generator.gumbel
Draw samples from a Gumbel distribution.
numpy.testing.tests.test_doctesting.check_random_directive
>>> 2+2
numpy.random.Generator.pareto
Draw samples from a Pareto II or Lomax distribution with
numpy.random.PCG64DXSM.jumped
Returns a new bit generator with the state jumped.
numpy.random.RandomState.beta
Draw samples from a Beta distribution.
numpy.random.RandomState.seed
Reseed a legacy MT19937 BitGenerator
numpy.random.RandomState.wald
Draw samples from a Wald, or inverse Gaussian, distribution.
numpy.random.RandomState.zipf
Draw samples from a Zipf distribution.
numpy.random.Generator.laplace
Draw samples from the Laplace or double exponential distribution with
numpy.random.Generator.poisson
Draw samples from a Poisson distribution.
numpy.random.Generator.shuffle
Modify an array or sequence in-place by shuffling its contents.
numpy.random.Generator.uniform
Draw samples from a uniform distribution.
numpy.random.Generator.weibull
Draw samples from a Weibull distribution.
numpy.random.PCG64DXSM.advance
advance(delta)
numpy.random.RandomState.gamma
Draw samples from a Gamma distribution.
numpy.random.RandomState.power
Draws samples in [0, 1] from a power distribution with positive
numpy.random.RandomState.randn
Return a sample (or samples) from the "standard normal" distribution.
numpy.random.Generator.binomial
Draw samples from a binomial distribution.
numpy.random.Generator.logistic
Draw samples from a logistic distribution.
numpy.random.Generator.rayleigh
Draw samples from a Rayleigh distribution.
numpy.random.Generator.vonmises
Draw samples from a von Mises distribution.
numpy.random.RandomState.gumbel
Draw samples from a Gumbel distribution.
numpy.random.RandomState.pareto
Draw samples from a Pareto II or Lomax distribution with
numpy.random.Generator.chisquare
Draw samples from a chi-square distribution.
numpy.random.Generator.dirichlet
Draw samples from the Dirichlet distribution.
numpy.random.Generator.geometric
Draw samples from the geometric distribution.
numpy.random.Generator.lognormal
Draw samples from a log-normal distribution.
numpy.random.Generator.logseries
Draw samples from a logarithmic series distribution.
numpy.random.RandomState.laplace
Draw samples from the Laplace or double exponential distribution with
numpy.random.RandomState.poisson
Draw samples from a Poisson distribution.
numpy.random.RandomState.shuffle
shuffle(x)
numpy.random.RandomState.uniform
Draw samples from a uniform distribution.
numpy.random.RandomState.weibull
Draw samples from a Weibull distribution.
numpy.random.Generator.standard_t
Draw samples from a standard Student's t distribution with `df` degrees
numpy.random.Generator.triangular
Draw samples from the triangular distribution over the
numpy.random.RandomState.binomial
Draw samples from a binomial distribution.
numpy.random.RandomState.logistic
Draw samples from a logistic distribution.
numpy.random.RandomState.rayleigh
Draw samples from a Rayleigh distribution.
numpy.random.RandomState.vonmises
Draw samples from a von Mises distribution.
numpy.random.Generator.exponential
Draw samples from an exponential distribution.
numpy.random.tests.test_generator_mt19937.TestIntegers.rfunc
Return random integers from `low` (inclusive) to `high` (exclusive), or
numpy.random.Generator.multinomial
Draw samples from a multinomial distribution.
numpy.random.RandomState.chisquare
Draw samples from a chi-square distribution.
numpy.random.RandomState.dirichlet
Draw samples from the Dirichlet distribution.
numpy.random.RandomState.geometric
Draw samples from the geometric distribution.
numpy.random.RandomState.get_state
get_state()
numpy.random.RandomState.lognormal
Draw samples from a log-normal distribution.
numpy.random.RandomState.logseries
Draw samples from a logarithmic series distribution.
numpy.random.RandomState.set_state
set_state(state)
numpy.polynomial.Hermite._fit
Least squares fit of Hermite series to data.
numpy.random.Generator.noncentral_f
Draw samples from the noncentral F distribution.
numpy.random.RandomState.standard_t
Draw samples from a standard Student's t distribution with `df` degrees
numpy.random.RandomState.triangular
Draw samples from the triangular distribution over the
numpy.polynomial.HermiteE._fit
Least squares fit of Hermite series to data.
numpy.polynomial.Laguerre._fit
Least squares fit of Laguerre series to data.
numpy.random.MT19937._legacy_seeding
_legacy_seeding(seed)
numpy.random.RandomState.exponential
Draw samples from an exponential distribution.
numpy.random.RandomState.multinomial
Draw samples from a multinomial distribution.
numpy.random.Generator.hypergeometric
Draw samples from a Hypergeometric distribution.
numpy.random.Generator.standard_gamma
Draw samples from a standard Gamma distribution.
numpy.random.RandomState.noncentral_f
Draw samples from the noncentral F distribution.
numpy.polynomial.Polynomial._fit
Least-squares fit of a polynomial to data.
numpy.random.Generator.standard_cauchy
Draw samples from a standard Cauchy distribution with mode = 0.
numpy.random.Generator.standard_normal
Draw samples from a standard Normal distribution (mean=0, stdev=1).
numpy.random.RandomState.hypergeometric
Draw samples from a Hypergeometric distribution.
numpy.random.RandomState.standard_gamma
Draw samples from a standard Gamma distribution.
numpy.random.Generator.negative_binomial
Draw samples from a negative binomial distribution.
numpy.random.RandomState.standard_cauchy
Draw samples from a standard Cauchy distribution with mode = 0.
numpy.random.RandomState.standard_normal
Draw samples from a standard Normal distribution (mean=0, stdev=1).
numpy.random.Generator.multivariate_normal
multivariate_normal(mean, cov, size=None, check_valid='warn',
numpy.random.RandomState.negative_binomial
Draw samples from a negative binomial distribution.
numpy.random.Generator.noncentral_chisquare
Draw samples from a noncentral chi-square distribution.
numpy.random.Generator.standard_exponential
Draw samples from the standard exponential distribution.
numpy.random.RandomState.noncentral_chisquare
Draw samples from a noncentral chi-square distribution.
numpy.random.RandomState.standard_exponential
Draw samples from the standard exponential distribution.
numpy.random.Generator.multivariate_hypergeometric
multivariate_hypergeometric(colors, nsample, size=None,
numpy.ma.tests.test_subclassing.SubArray
ndarray(shape, dtype=float, buffer=None, offset=0,
numpy.core.tests.test_function_base.PhysicalQuantity2
ndarray(shape, dtype=float, buffer=None, offset=0,
numpy.lib.tests.test_stride_tricks.SimpleSubClass
ndarray(shape, dtype=float, buffer=None, offset=0,
numpy.ma.tests.test_subclassing.ComplicatedSubArray
ndarray(shape, dtype=float, buffer=None, offset=0,
numpy.lib.tests.test_stride_tricks.VerySimpleSubClass
ndarray(shape, dtype=float, buffer=None, offset=0,
numpy.core.tests.test_multiarray.TestArrayPriority.Foo
ndarray(shape, dtype=float, buffer=None, offset=0,
numpy.core.tests.test_multiarray.TestArrayPriority.Bar
ndarray(shape, dtype=float, buffer=None, offset=0,
a_list = [1,2,3,4]
print(type(a_list))
<class 'list'>
a_np = np.array(a_list) # converting a list to a numpy array
print(type(a_np))
<class 'numpy.ndarray'>
a_list * 2
[1, 2, 3, 4, 1, 2, 3, 4]
a_np * 2
array([2, 4, 6, 8])
b_np = np.array([1,1,1,1])
a_np + b_np #elementwise operation
array([2, 3, 4, 5])
a_list + [1,1,1,1] #concatenation
[1, 2, 3, 4, 1, 1, 1, 1]
np.arange(5)
array([0, 1, 2, 3, 4])
new_np = np.arange(10)
new_np
array([0, 1, 2, 3, 4, 5, 6, 7, 8, 9])
#Calculate some basic stats on numpy arrays
#new_np.max()
#new_np.min()
new_np.mean()
4.5
#Zero matrix or Array
#No more list comprehension to initialize a zero matrix
a_zeros = np.zeros((3,4))
a_zeros
array([[0., 0., 0., 0.],
[0., 0., 0., 0.],
[0., 0., 0., 0.]])
type(a_zeros)
numpy.ndarray
a_ones = np.ones((5,5))
a_ones
array([[1., 1., 1., 1., 1.],
[1., 1., 1., 1., 1.],
[1., 1., 1., 1., 1.],
[1., 1., 1., 1., 1.],
[1., 1., 1., 1., 1.]])
#Identity Matrix
np.identity(5) #np.eye(5)
array([[1., 0., 0., 0., 0.],
[0., 1., 0., 0., 0.],
[0., 0., 1., 0., 0.],
[0., 0., 0., 1., 0.],
[0., 0., 0., 0., 1.]])
new_np
array([0, 1, 2, 3, 4, 5, 6, 7, 8, 9])
new_np.T #transpose
array([0, 1, 2, 3, 4, 5, 6, 7, 8, 9])
#Change dimensions of array
new_np.reshape(2,5)
array([[0, 1, 2, 3, 4],
[5, 6, 7, 8, 9]])
Note: Reshape dimensions must match with original size of array
Cannot reshape an array of length 20 into (2,5)
new_np.reshape(2,5).T
array([[0, 5],
[1, 6],
[2, 7],
[3, 8],
[4, 9]])
np.random.random?
np.random.randint?
np.random.randint(1,5,10)
array([3, 2, 4, 2, 4, 3, 3, 4, 2, 1])
##Multiple operations in a single line
t_mat = np.random.randint(1,5,20).reshape(4,5)
t_mat
array([[2, 1, 1, 1, 2],
[4, 2, 4, 1, 1],
[1, 3, 3, 2, 1],
[4, 1, 4, 4, 3]])
t_mat.shape
(4, 5)
Indexing and slicing#
t_mat[0] #extracts the first row
array([2, 1, 1, 1, 2])
t_mat[0][0] #extracts the first element
2
t_mat[3][2]
4
t_mat[3,2]
4
t_mat[:,:]
array([[2, 1, 1, 1, 2],
[4, 2, 4, 1, 1],
[1, 3, 3, 2, 1],
[4, 1, 4, 4, 3]])
t_mat[1,:] # same as t_mat[1] ->gives the row at row index 1
array([4, 2, 4, 1, 1])
t_mat [:, 1] # column at column index 1
array([1, 2, 3, 1])
t_mat[1:3,1:3] # lower index included upper index excluded
array([[2, 4],
[3, 3]])
t_mat[:2,2:]
array([[1, 1, 2],
[4, 1, 1]])
Numpy Universal Functions#
np.sin(t_mat)
array([[ 0.90929743, 0.84147098, 0.84147098, 0.84147098, 0.90929743],
[-0.7568025 , 0.90929743, -0.7568025 , 0.84147098, 0.84147098],
[ 0.84147098, 0.14112001, 0.14112001, 0.90929743, 0.84147098],
[-0.7568025 , 0.84147098, -0.7568025 , -0.7568025 , 0.14112001]])
np.sqrt(t_mat)
array([[1.41421356, 1. , 1. , 1. , 1.41421356],
[2. , 1.41421356, 2. , 1. , 1. ],
[1. , 1.73205081, 1.73205081, 1.41421356, 1. ],
[2. , 1. , 2. , 2. , 1.73205081]])
np.lookfor("hyperbolic")
Show code cell output
Search results for 'hyperbolic'
-------------------------------
numpy.cosh
Hyperbolic cosine, element-wise.
numpy.sinh
Hyperbolic sine, element-wise.
numpy.tanh
Compute hyperbolic tangent element-wise.
numpy.arccosh
Inverse hyperbolic cosine, element-wise.
numpy.arcsinh
Inverse hyperbolic sine element-wise.
numpy.arctanh
Inverse hyperbolic tangent element-wise.
numpy.ma.cosh
Hyperbolic cosine, element-wise.
numpy.ma.sinh
Hyperbolic sine, element-wise.
numpy.ma.tanh
Compute hyperbolic tangent element-wise.
numpy.ma.arccosh
Inverse hyperbolic cosine, element-wise.
numpy.ma.arcsinh
Inverse hyperbolic sine element-wise.
numpy.ma.arctanh
Inverse hyperbolic tangent element-wise.
numpy.random.Generator.standard_t
Draw samples from a standard Student's t distribution with `df` degrees
numpy.random.RandomState.standard_t
Draw samples from a standard Student's t distribution with `df` degrees
Conditonal Logic with Numpy#
#Boolean Operations
# == , < , > , =< , >=
x = 3
x == 5
False
t_mat
array([[2, 1, 1, 1, 2],
[4, 2, 4, 1, 1],
[1, 3, 3, 2, 1],
[4, 1, 4, 4, 3]])
x == t_mat
array([[False, False, False, False, False],
[False, False, False, False, False],
[False, True, True, False, False],
[False, False, False, False, True]])
t_mat % 2 == 0
array([[ True, False, False, False, True],
[ True, True, True, False, False],
[False, False, False, True, False],
[ True, False, True, True, False]])
With a boolean array (array with True/False), you can index with it to select particular values.
Here we can select all multiples of 2:
t_mat[t_mat % 2 == 0]
array([2, 2, 4, 2, 4, 2, 4, 4, 4])
Here we set all 4’s to 0:
t_mat[t_mat == 4] = 0
t_mat
array([[2, 1, 1, 1, 2],
[0, 2, 0, 1, 1],
[1, 3, 3, 2, 1],
[0, 1, 0, 0, 3]])
Matplotlib –> visualization/plotting library#
plt.plot([2,6,4,9])
[<matplotlib.lines.Line2D at 0x7fa020fac3a0>]
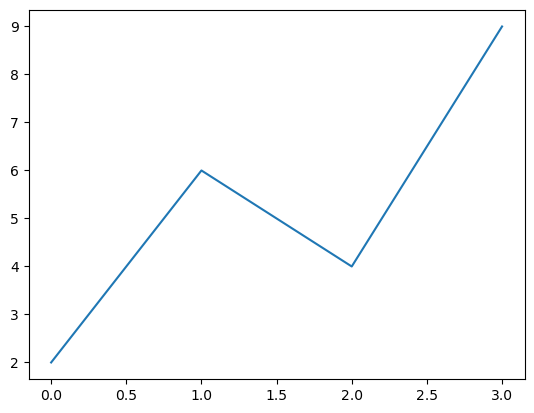
x = np.arange(10)
y = x**2
plt.plot(x,y)
[<matplotlib.lines.Line2D at 0x7fa063b75d00>]
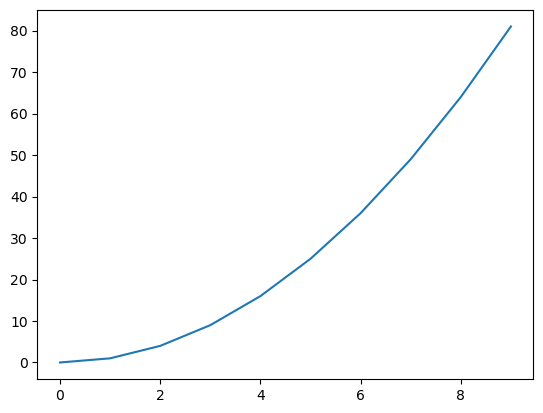
plt.plot?
x = np.arange(10)
y = x**2
plt.plot(x,y, 'mH')
plt.xlabel("integer values from 0-9")
plt.ylabel("x squared")
Text(0, 0.5, 'x squared')
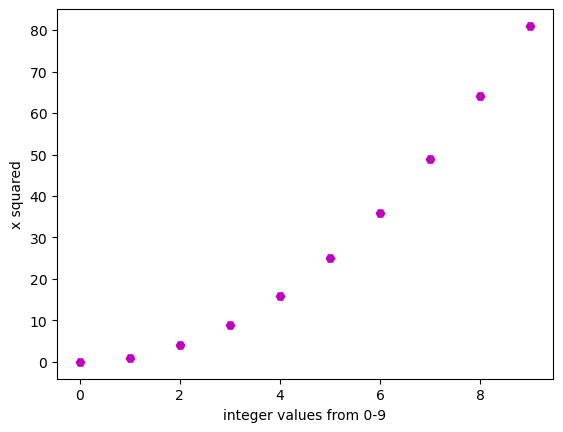
plt.bar(["cats","dogs","birds","fishes"], [3,4,2,7], width=0.75)
plt.xlabel("pets")
plt.ylabel("no. of pets")
Text(0, 0.5, 'no. of pets')
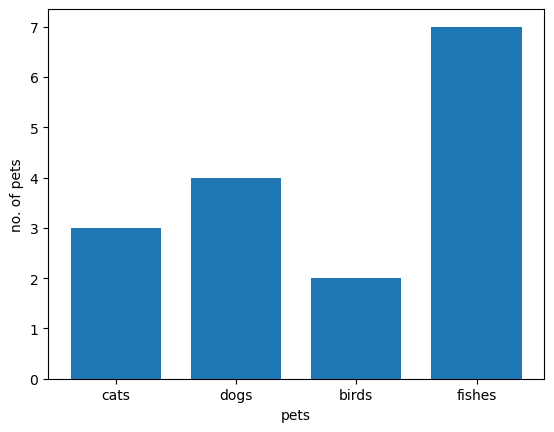
plt.bar?
plt.scatter(np.arange(10),np.random.randint(3,8,10), c = "red", marker = "H")
<matplotlib.collections.PathCollection at 0x7fa063bb59a0>
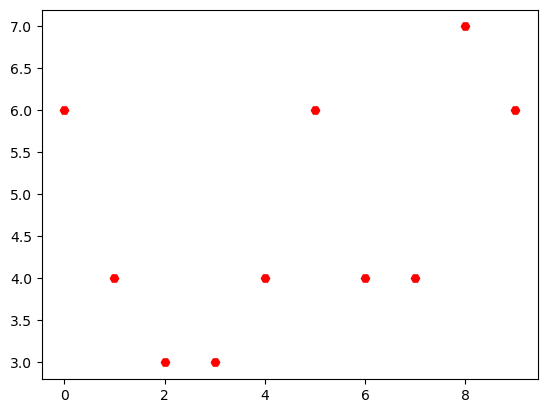
plt.scatter?
#subplots
plt.figure(figsize=(9,3))
plt.subplot(131)
plt.bar(["cats","dogs","birds","fishes"], [3,4,2,7], width=0.75)
plt.xlabel("pets")
plt.ylabel("no. of pets")
plt.subplot(133)
plt.scatter(np.arange(10),np.random.randint(3,8,10), c = "red", marker = "H")
plt.subplot(132)
x = np.arange(10)
y = x**2
plt.plot(x,y, 'mH')
plt.xlabel("integer values from 0-9")
plt.ylabel("x squared")
plt.suptitle("Different Plots in sublot format")
plt.show()
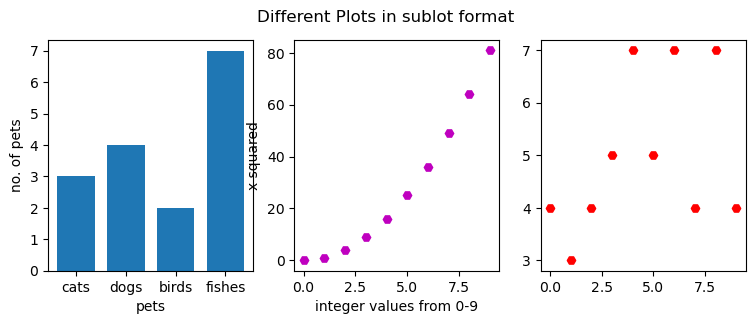
plt.subplot?
##Functions for plotting
def bar_plot():
plt.bar(["cats","dogs","birds","fishes"], [3,4,2,7], width=0.75)
plt.xlabel("pets")
plt.ylabel("no. of pets")
def line_plot():
x = np.arange(10)
y = x**2
plt.plot(x,y, 'mH')
plt.xlabel("integer values from 0-9")
plt.ylabel("x squared")
def scatter_plot():
plt.scatter(np.arange(10),np.random.randint(3,8,10), c = "red", marker = "H")
plt.figure(figsize=(9,3))
plt.subplot(131)
bar_plot()
plt.subplot(133)
scatter_plot()
plt.subplot(132)
line_plot()
plt.suptitle("Different Plots in sublot format")
plt.show()
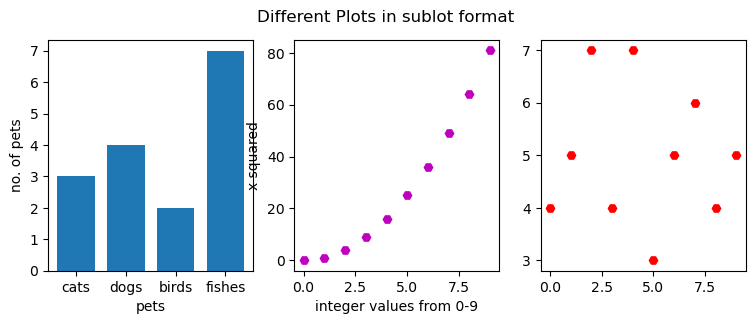